#include <iostream>
#include <string>
using namespace std;
// 이중 연결 리스트의 노드 정의
class Node {
public:
int playerNumber;
string playerName;
Node* prev;
Node* next;
Node(int num, const string name) : playerNumber(num), playerName(name), prev(nullptr), next(nullptr) {}
};
// 해시 테이블 클래스 정의
class HashTable {
private:
static const int TABLE_SIZE = 10; // 해시 테이블 크기
Node** table; // 해시 테이블 배열
public:
HashTable() {
table = new Node * [TABLE_SIZE];
for (int i = 0; i < TABLE_SIZE; ++i) {
table[i] = nullptr; // 초기화
}
}
~HashTable() {
for (int i = 0; i < TABLE_SIZE; ++i) {
Node* current = table[i];
while (current) {
Node* toDelete = current;
current = current->next;
delete toDelete;
}
}
delete[] table;
}
// 삽입 함수
void set(int playerNumber, const string& playerName) {
int index = playerNumber % TABLE_SIZE;
Node* newNode = new Node(playerNumber, playerName);
// 리스트의 맨 앞에 추가
if (table[index]) {
newNode->next = table[index];
table[index]->prev = newNode;
}
table[index] = newNode;
}
// 검색 함수
string get(int playerNumber) {
int index = playerNumber % TABLE_SIZE;
Node* current = table[index];
while (current) {
if (current->playerNumber == playerNumber) {
return current->playerName; // 선수 이름 반환
}
current = current->next;
}
return "선수를 찾을 수 없습니다."; // 선수 없음
}
// 삭제 함수
void remove(int playerNumber) {
int index = playerNumber % TABLE_SIZE;
Node* current = table[index];
while (current) {
if (current->playerNumber == playerNumber) {
if (current->prev) {
current->prev->next = current->next;
}
else {
table[index] = current->next; // 첫 번째 노드 삭제
}
if (current->next) {
current->next->prev = current->prev;
}
delete current;
return;
}
current = current->next;
}
}
};
복잡 스럽네요.. DoublyLinkedList를 가져와서 구현하려고 하니까. 일단.. 안되가지고. 애초애 javascript는 잘 모르겠지만...
string을 넘겨주고 있는데. 만들어준 DoublyLinkedList는 호환되지 않네요..;;
일단. 방법을 좀 찾고 싶었는데.... 흠.. 너무 꼬여버려서. 뭔지 모르겠네요.. 흠.. 스터디에 끌려가서 큰일이네요. 빨리 끝내야 하는데. 하면서 하니까>
#include <iostream>
#include "Hash.h"
using namespace std;
int main()
{
HashTable hash;
hash.set(1, "이운재");
hash.set(4, "최진철");
hash.set(20, "홍명보");
hash.set(6, "유상철");
hash.set(22, "송종국");
hash.set(21, "박지성");
hash.set(5, "김남일");
hash.set(10, "이영표");
hash.set(8, "최태욱");
hash.set(9, "설기현");
hash.set(14, "이천수");
cout << "1번 선수: " << hash.get(1) << endl;
hash.remove(1);
cout << "1번 선수: " << hash.get(1) << endl;
cout << "21번 선수: " << hash.get(21) << endl;
return 0;
}
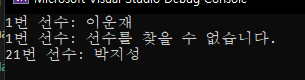
DoublyLinkedList를 구현하고 디버깅하면서 해시테이블을 만드는 방법도 좋을 것 같습니다.
지금처럼 내부에 직접구현해서 하는 것도 현재 상황에서 최선을 다하신 것 같아서 보기좋습니다!
고생하셨습니다😊
답글
빠타박스
2024.10.04감사합니다!