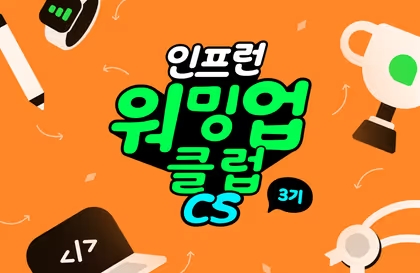
인프런 워밍업 클럽 스터디 3기 - 자료구조와 알고리즘 -2주차 미션-
2개월 전
1. 재귀함수에서 기저조건을 만들지 않거나 잘못 설정했을 때 어떤 문제가 발생할 수 있나요?
스택 오버플로가 발생합니다.
콜스택에 스택 프레임이 쌓이는데, 기저조건을 만들지 않으면 함수가 무제한 호출되며, 스택 프레임도 무제한으로 쌓이게 됩니다.
콜스택 메모리의 잉여 용량을 초과하면 프로세스가 OS에 의해 강제 종료되는데 이를 스택 오버플로라고 합니다.
2. 0부터 입력 n까지 홀수의 합을 더하는 재귀함수를 만들어보세요.
function sumOdd(n) {
if (n <= 0) return 0;
// 현재 숫자가 홀수면 더하고, 짝수면 그냥 넘긴다
if (n % 2 !== 0) {
return n + sumOdd(n - 1);
} else {
return sumOdd(n - 1);
}
}
console.log(sumOdd(10)); // 25
3. 다음 코드는 매개변수로 주어진 파일 경로(.는 현재 디렉토리)에 있는 하위 모든 파일과 디렉토리를 출력하는 코드입니다. 다음 코드를 재귀 함수를 이용하는 코드로 변경해보세요.
const fs = require("fs"); // 파일을 이용하는 모듈
const path = require("path"); // 폴더와 파일의 경로를 지정해주는 모듈
function traverseDirectory1(directory){
const stack = [directory]; // 순회해야 할 디렉토리를 저장할 스택
while (stack.length > 0) { // 스택이 빌 때까지 반복
const currentDir = stack.pop(); // 현재 디렉토리
const files = fs.readdirSync(currentDir); // 인자로 주어진 경로의 디렉토리에 있는 파일 or 디렉토리들
for (const file of files) { // 현재 디렉토리의 모든 파일 or 디렉토리 순회
const filePath = path.join(currentDir, file); // directory와 file을 하나의 경로로 합쳐줌
const fileStatus= fs.statSync(filePath); // 파일정보 얻기
if (fileStatus.isDirectory()) { // 해당 파일이 디렉토리라면
console.log('디렉토리:', filePath);
stack.push(filePath);
} else { // 해당 파일이 파일이라면
console.log('파일:', filePath);
}
}
}
}
traverseDirectory1("."); // 현재 경로의 모든 하위 경로의 파일, 디렉토리 출력
const fs = require("fs"); // 파일을 이용하는 모듈
const path = require("path"); // 폴더와 파일의 경로를 지정해주는 모듈
function recursiveTraverseDirectory(directory) {
const files = fs.readdirSync(directory);
for (const file of files) {
const filePath = path.join(directory, file);
const fileStatus = fs.statSync(filePath);
if (fileStatus.isDirectory()) {
console.log('Directory:', filePath);
recursiveTraverseDirectory(filePath);
} else {
console.log('File:', filePath);
}
}
}
recursiveTraverseDirectory("."); // 현재 경로의 모든 하위 경로의 파일, 디렉토리 출력
댓글을 작성해보세요.